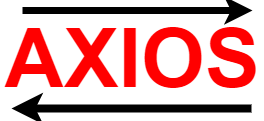
What is Axios
Axios is a promise based HTTP client for browser and node.js; it can be used on server side and client side (Browser) to interact with API (Application Programming Interface to send request from the browser and node.js server to other API and get a response).
On client side(Browser) Axios uses XMLHttpRequests and On server-side it uses http module
Installing Axios in your node.js project
$ npm install axios
How to use axios get request
const axios = require(‘axios’);
let userID = ‘123456’;
axios.get(`/userInfo?id=${userID}`).
then(response=>{
console.log(response)
}).catch(error=>{
console.log(error)
});
Using async/await
async function getUserInfo(){
try{
let userInfo = await axios.get(““/userInfo?id=${userID}`);
}catch(error){
console.log(error)
}
}
How to use axios post request
axios.post(‘/userInfo’, {
firstName: ‘FName’,
lastName: ‘LName’,
dob: ‘06/15/2001’
}).then(response=> {
console.log(response);
}).catch(error=> {
console.log(error);
});
How to use axios delete request.
axios.delete(‘/userInfo/’+{userID}).then(response=>{
console.log(response);
}).catch(error=>{
console.log(error);
});
Axios get request for remote image in node.js.
axios({
method: ‘get’,
url: ‘https://images.app.goo.gl/3g4ez2auLnyoYG1u8’,
responseType: ‘stream’
}).then(response=> {
response.data.pipe(fs.createWriteStream(‘forest.jpg’))
}).catch(error=>{
console.log(error)
});
To send a file with Axios in node.js.
To send file with Axios in node.js
let url = `API_URL` //API URl where you post filelet fileRecievedFromClientSide = req.file;
let buffer = fs.readFileSync(fileRecievedFromClientSide.path);
let form = new FormData();
form.append(‘xlsx’, buffer, fileRecievedFromClientSide.originalname);
Axios.post(url, form, {
‘maxContentLength’: Infinity,
‘maxBodyLength’: Infinity,
headers: {
‘Content-Type’: `multipart/form-data,
boundary=${form._boundary}`,
}
}).then(response => {
console.log(response)
}).catch(error => {
console.log(error)
});
Hope you would love this summary!